前言
常用的Web Server是Nginx, Apache等。Go可以几行代码就可以创建Web Serve。
这里使用net.http包,十分简单就可以实现一个建议的http服务器。
从简单到复杂,分成几个个版本。
参考官方net/http文档
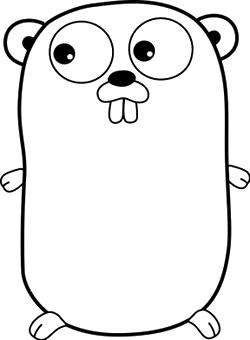
版本1
文件server.go
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| package main
import ( "net/http" "io" ) func main() { http.HandleFunc("/", HelloServer) http.ListenAndServe(":5000", nil) }
func HelloServer(w http.ResponseWriter, req * http.Request) { io.WriteString(w, "hello, world!") }
|
运行 go run server.go
测试访问localhost:5000
时,返回hello, world
版本2
对代码进行简单修改
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| package main
import ( "net/http" "io" ) func main() { http.HandleFunc("/", HelloServer) http.HandleFunc("/test", TestServer) http.ListenAndServe(":5000", nil) }
func HelloServer(w http.ResponseWriter, req * http.Request) { io.WriteString(w, "hello, world!") }
func TestServer(w http.ResponseWriter, req * http.Request) { io.WriteString(w, "Url: " + req.URL.Path) }
|
访问localhost:5000
时,返回hello, world
访问localhost:5000/test
时,返回访问路径Url:/test
版本3
增加路由判断
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| package main
import ( "net/http" ) func main() { http.HandleFunc("/", handle) http.ListenAndServe(":5000", nil) }
func handle(w http.ResponseWriter, req * http.Request) { w.Header().Set("Content-Type", "text/plain") url := req.URL.Path if url == "/" { w.Write([]byte("hello, world!.\n")) }else{ w.Write([]byte("Url:" + url + ".\n")) } }
|
通过handle
方法,统一接受所有请求,针对不同Url,返回不同数据
版本4
通过http.NewServeMux()
增加连接超时时间
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35
| package main
import ( "log" "net/http" "time" )
func main(){ mux := http.NewServeMux() mux.Handle("/", &MyHandle{}) mux.HandleFunc("/say", sayBye)
srv := &http.Server{ Addr: ":5000", WriteTimeout:time.Second * 3, Handler:mux, } log.Println("Service start :5000") log.Fatal(srv.ListenAndServe()) }
func HandleFunc(w http.ResponseWriter, r *http.Request){ w.Write([]byte("success")) }
type MyHandle struct{}
func (*MyHandle) ServeHTTP(w http.ResponseWriter, r *http.Request){ w.Write([]byte("success now")) }
func sayBye(w http.ResponseWriter, r *http.Request){ w.Write([]byte("bye bye, this is v2")) }
|